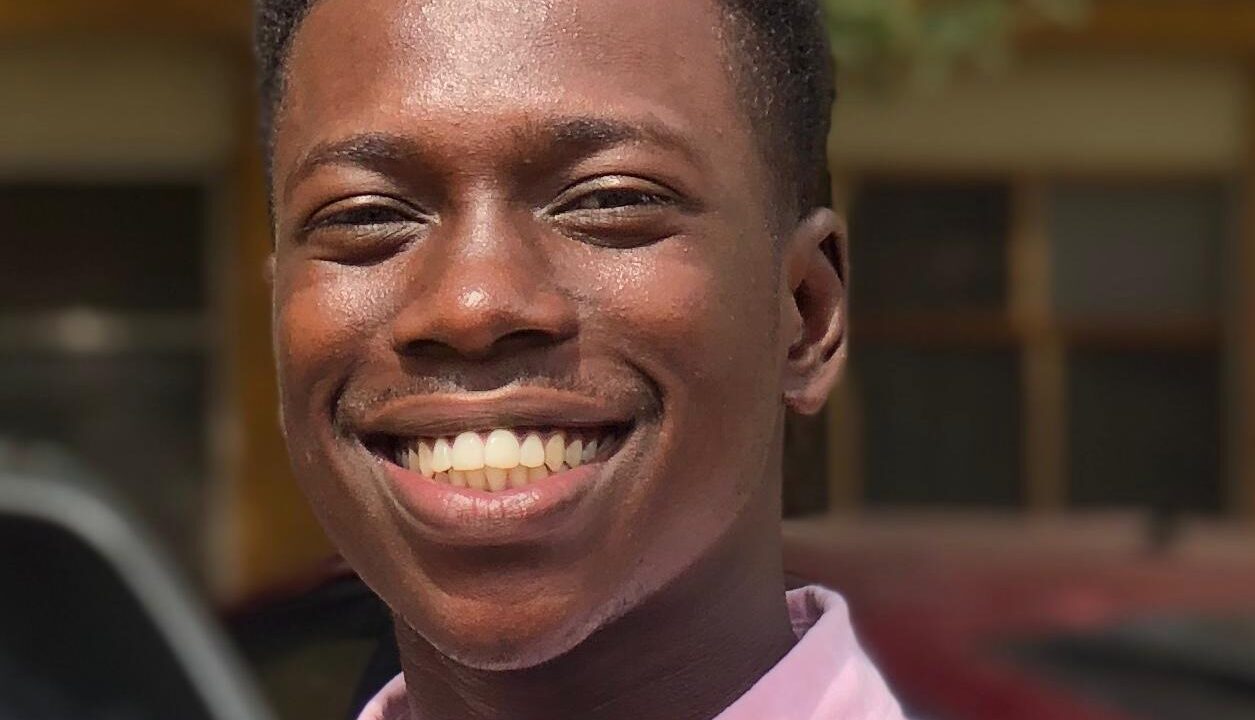
Machine Learning has revolutionized various industries by enabling intelligent systems to learn from data and make accurate predictions or decisions. However, traditional centralized machine learning approaches often require data to be collected and stored in a central location, raising privacy and security concerns, especially when dealing with sensitive data such as medical records or financial information.
Federated Learning is an emerging machine learning paradigm that addresses these concerns by enabling the training of machine learning models on decentralized data across multiple devices or organizations, without the need to share or transfer raw data. This approach not only preserves data privacy but also reduces communication costs and enables collaborative model training.
In this article, we’ll dive into the world of Federated Learning, explore its key principles and algorithms, and provide a practical implementation using PyTorch, a popular deep learning library. We’ll train an image classification model on the CIFAR-10 dataset, which consists of 60,000 32×32 colour images across 10 classes, such as airplanes, dogs, and horses.
Understanding Federated Learning
Federated Learning is a distributed machine learning approach where the model training occurs locally on each device or organization (known as clients), and the updates are then aggregated on a central server. This process is repeated iteratively until the desired model performance is achieved.
The key principles of Federated Learning are:
⦁ Privacy Preservation: By training models locally on each client’s data, sensitive information never leaves the device, ensuring data privacy.
⦁ Collaborative Learning: Multiple clients contribute to the training process, enabling the model to learn from diverse data sources and improve its generalization capabilities.
⦁ Efficient Communication: Only model updates (e.g., gradients or weight updates) are transmitted between clients and the server, reducing communication overhead compared to sharing raw data.
Federated Learning is particularly relevant in scenarios where data privacy is a critical concern, such as healthcare, finance, and Internet of Things (IoT) devices. For example, in the healthcare domain, patient records are highly sensitive and subject to strict privacy regulations. Federated Learning enables training machine learning models on these distributed patient records without compromising data privacy. Similarly, in the financial sector, customer data and transaction records are confidential. Federated Learning allows banks and financial institutions to collaboratively train models without sharing their customers’ sensitive data.
Federated Learning Algorithms
Two popular algorithms used in Federated Learning are FedAvg (Federated Averaging) and FedSGD (Federated Stochastic Gradient Descent).
FedAvg is a simple yet effective algorithm that enables the training of machine learning models in a decentralized manner, where the data is distributed across multiple clients (devices, organizations, or data silos). The key idea behind FedAvg is to leverage the computational resources of the clients to perform local training on their respective data, and then aggregate the locally trained models at a central server to obtain a global model.
The FedAvg algorithm follows these steps:
⦁ Initialization: The process begins with a central server initializing a global model with random weights or pre-trained weights from a different dataset.
⦁ Distribution of the Global Model: The server sends the current global model weights to each participating client.
⦁ Local Training: Each client trains the received global model on their local data for a specified number of epochs or iterations, using their local optimizer and loss function. This local training step is performed independently and in parallel across all clients.
⦁ Local Model Updates: After local training, each client computes the updates to the model weights based on their local data and training process. These updates can be in the form of gradients, model weight differences, or the entire updated model weights.
⦁ Aggregation of Local Updates: The clients send their local model updates back to the central server.
⦁ Averaging of Local Updates: The server aggregates the local model updates from all participating clients. In the original FedAvg algorithm, this aggregation is done by taking a weighted average of the client model updates, where the weights are typically proportional to the size of the client’s dataset. This averaging step ensures that the global model captures the diversity of data across different clients while mitigating the effects of non-IID (non-independent and identically distributed) data distributions.
⦁ Global Model Update: The server updates the global model weights by applying the averaged updates from the clients.
⦁ Iteration: Steps 2-7 are repeated for a pre-defined number of communication rounds or until the global model converges to a satisfactory performance.
FedSGD is a variant of FedAvg. The only difference is that it incorporates momentum and adaptive learning rate techniques, potentially leading to faster convergence and better performance.
Implementing Federated Learning with PyTorch
Let’s dive into a practical implementation of Federated Learning using PyTorch. We’ll simulate a decentralized environment with multiple clients and a central server, and train an image classification model on the CIFAR-10 dataset using the FedAvg algorithm.
Setting up the Environment
First, we’ll import the necessary libraries:
import numpy as np
import torch
import torch.nn as nn
import torch.optim as optim
from torch.utils.data import DataLoader
from torchvision import datasets, transforms
import copy
We’ll then define some helper functions. The first helper function we’ll need, the partition_data function, simulates a non-IID data distribution by first shuffling the dataset indices randomly. Then, splits the shuffled indices into shards, where each shard represents the data for a single client. It employs the torch.utils.data. Subset class to create a subset of the original dataset for each client based on their respective indices.
Helper function to simulate non-IID data distribution
def partition_data(dataset, num_clients):
# Partitioning the data into shards for each client
data_len = len(dataset)
indices = list(range(data_len))
split_size = data_len // num_clients
# Shuffling the data indices
np.random.seed(42)
np.random.shuffle(indices)
# Partitioning the indices into shards
client_data = []
for i in range(num_clients):
start = i * split_size
end = start + split_size
client_data.append(torch.utils.data.Subset(dataset, indices[start:end]))
return client_data
The next helper function we’ll create is the evaluate function which calculates the accuracy of the given model on the test dataset. It sets the model to evaluation mode, iterates over the test data loader, computes the predicted labels, and calculates the overall accuracy by comparing the predicted labels with the ground truth labels.
Helper function to evaluate the global model
def evaluate(model, test_loader):
model.eval()
correct = 0
total = 0
with torch.no_grad():
for data, target in test_loader:
output = model(data)
_, predicted = torch.max(output.data, 1)
total += target.size(0)
correct += (predicted == target).sum().item()
accuracy = 100 * correct / total
return accuracy
With these helper functions, we can proceed with the implementation of the Federated Learning process using the FedAvg algorithm.
Defining the Model and Clients
Next, we’ll define a simple convolutional neural network (CNN) for image classification and create a Client class to represent each participating device or organization. In the CNN class, we define a simple convolutional neural network architecture with two convolutional layers, two max-pooling layers, and two fully-connected layers. The forward method defines the forward pass of the network, taking an input tensor x and returning the output logits.
Define the CNN model
class CNN(nn.Module):
def init(self):
super(CNN, self).init()
self.conv1 = nn.Conv2d(3, 32, kernel_size=3, padding=1)
self.relu = nn.ReLU()
self.maxpool = nn.MaxPool2d(kernel_size=2, stride=2)
self.conv2 = nn.Conv2d(32, 64, kernel_size=3, padding=1)
self.fc1 = nn.Linear(64 * 8 * 8, 128)
self.fc2 = nn.Linear(128, 10)
def forward(self, x):
out = self.conv1(x)
out = self.relu(out)
out = self.maxpool(out)
out = self.conv2(out)
out = self.relu(out)
out = self.maxpool(out)
out = out.view(-1, 64 * 8 * 8)
out = self.relu(self.fc1(out))
out = self.fc2(out)
return out
In the Client class, we initialize an instance of the CNN model, an optimizer (Stochastic Gradient Descent), a loss function (Cross-Entropy Loss), and a data loader for the client’s training data. We then create a train method to perform local training on the client’s data for the specified number of epochs. For each epoch, it iterates over the training data loader, computes the model output and loss, performs backpropagation, and updates the model parameters using the optimizer.
Define the Client class
class Client(object):
def init(self, train_data, batch_size, lr, epochs):
self.train_loader = DataLoader(train_data, batch_size=batch_size, shuffle=True)
self.model = CNN()
self.optimizer = optim.SGD(self.model.parameters(), lr=lr)
self.epochs = epochs
self.criterion = nn.CrossEntropyLoss()
def train(self):
for epoch in range(self.epochs):
for batch_idx, (data, target) in enumerate(self.train_loader):
self.optimizer.zero_grad()
output = self.model(data)
loss = self.criterion(output, target)
loss.backward()
self.optimizer.step()
return self.model.state_dict()
After training, the train method returns the current state dictionary of the model, containing the learned weights and biases. This state dictionary can be sent to the server for aggregation in the Federated Learning process. The server can then coordinate the Federated Learning process by distributing the global model to the clients, collecting their updated model states, and aggregating them using the FedAvg algorithm.
Federated Averaging (FedAvg) Implementation
Now, let’s implement the FedAvg algorithm by defining a Server class and the training loop. In this class, we’ll create three functions: an init method, federated_averaging function, and an average_weights function. The init method initializes the server with the list of clients, the test data loader, the number of rounds, the learning rate, the number of epochs, and an instance of the CNN model as the global model.
class Server(object):
def init(self, clients, test_loader, num_rounds, lr, epochs):
self.clients = clients
self.test_loader = test_loader
self.num_rounds = num_rounds
self.lr = lr
self.epochs = epochs
self.global_model = CNN()
The federated_averaging method implements the FedAvg algorithm. It iterates over the specified number of rounds. In each round, it performs the following steps:
⦁ Iterate over the clients
⦁ Send the current global model weights to the client
⦁ Train the client model locally using the train method
⦁ Collect the updated client model weights
⦁ Aggregate the client model weights using the average_weights method
⦁ Load the averaged weights into the global model
⦁ Evaluate the global model on the test data loader and print the accuracy
def federated_averaging(self):
for round in range(self.num_rounds):
client_weights = []
for client in self.clients:
# Send the global model to the client
client.model.load_state_dict(self.global_model.state_dict())
# Train the client model
client_weights.append(client.train())
# Aggregate the client model weights self.global_model.load_state_dict(self.average_weights(client_weights))
# Evaluate the global model
accuracy = evaluate(self.global_model, self.test_loader)
print(f"Round {round}, Accuracy: {accuracy:.4f}")
The average_weights function implements the weight averaging step, which can be a simple arithmetic mean or a more sophisticated aggregation technique. In this example, it takes a list of client model weights and averages them to obtain the new global model weights. It does that by:
⦁ Initialize the avg_weights dictionary with the weights from the first client.
⦁ Calculate the number of clients.
⦁ Iterate over the keys (weight tensors) in the avg_weights dictionary.
⦁ Initialize a tensor to store the sum of weights for the current weight tensor.
⦁ Sum the corresponding weight tensors from all clients.
⦁ Average the summed weights by dividing by the number of clients.
⦁ Update the avg_weights dictionary with the averaged weight tensor.
⦁ Return the avg_weights dictionary containing the averaged weights.
def average_weights(self, client_weights):
# Initialize the averaged weights
avg_weights = copy.deepcopy(client_weights[0])
# Get the number of clients
num_clients = len(client_weights)
# Iterate over the model weights
for w in avg_weights.keys():
# Initialize the sum of weights
weight_sum = torch.zeros_like(avg_weights[w])
# Sum the weights from all clients
for client_weight in client_weights:
weight_sum += client_weight[w]
# Average the weights
avg_weights[w] = weight_sum / num_clients
return avg_weights
Running the Federated Learning Process
Finally, we can set up the clients, server, and run the federated learning process:
We first load the CIFAR-10 dataset from the torchvision.datasets module. We define a transformation pipeline using transforms.Compose to normalize the input images.
Load and partition the dataset
transform = transforms.Compose([
transforms.ToTensor(),
transforms.Normalize((0.5, 0.5, 0.5), (0.5, 0.5, 0.5))
])
train_dataset = datasets.CIFAR10(root='data', train=True, download=True, transform=transform)
test_dataset = datasets.CIFAR10(root='data', train=False, download=True, transform=transform)
Next, we partition the training dataset into non-IID shards for each client using the partition_data helper function, as before.
Partition dataset into non-IID shards
num_clients = 10
client_data = partition_data(train_dataset, num_clients)
We then create a list of Client instances, each initialized with their respective data shard, batch size, learning rate, and number of epochs.
Create the clients
clients = [Client(data, batch_size=32, lr=0.01, epochs=1) for data in client_data]
We create a data loader for the test dataset and instantiate the Server class with the list of clients, the test data loader, the number of rounds, learning rate, and number of epochs.
Create the server
test_loader = DataLoader(test_dataset, batch_size=64, shuffle=False)
server = Server(clients, test_loader, num_rounds=20, lr=0.01, epochs=1)
Finally, we call the federated_averaging method on the server instance to run the Federated Learning process for 20 rounds using the FedAvg algorithm.
Run the federated learning process
server.federated_averaging()
Throughout the training process, the global model’s accuracy on the CIFAR-10 test dataset will be printed after each round, allowing us to monitor the model’s performance on the image classification task.
Output :
Downloading https://www.cs.toronto.edu/~kriz/cifar-10-python.tar.gz to data/cifar-10-python.tar.gz
100%|██████████| 170498071/170498071 [00:03<00:00, 43770747.65it/s]
Extracting data/cifar-10-python.tar.gz to data
Files already downloaded and verified
Round 0, Accuracy: 58.7500
Round 1, Accuracy: 67.5800
Round 2, Accuracy: 72.2800
Round 3, Accuracy: 75.2000
Round 4, Accuracy: 77.5100
Round 5, Accuracy: 78.9400
Round 6, Accuracy: 81.8400
Round 7, Accuracy: 82.9600
Round 8, Accuracy: 84.4400
Round 9, Accuracy: 86.3500
Round 10, Accuracy: 87.3900
Round 11, Accuracy: 88.2200
Round 12, Accuracy: 89.0200
Round 13, Accuracy: 90.2800
Round 14, Accuracy: 91.1100
Round 15, Accuracy: 91.5100
Round 16, Accuracy: 92.3400
Round 17, Accuracy: 92.7700
Round 18, Accuracy: 94.0700
Round 19, Accuracy: 94.1600
Conclusion
Federated Learning is a powerful paradigm that enables collaborative machine learning while preserving data privacy. By implementing the FedAvg algorithm using PyTorch, we’ve demonstrated how to train a simple image classification model in a decentralized manner, simulating a real-world scenario with multiple clients contributing to the training process.
However, this is just the tip of the iceberg. Federated Learning opens up exciting research avenues and practical applications in various domains, including healthcare, finance, and smart devices. As the field continues to evolve, we can expect to see more advanced algorithms, privacy-enhancing techniques, and efficient communication protocols for Federated Learning.
By understanding the principles of Federated Learning and gaining practical experience through implementations like the one demonstrated in this article, you’ll be well-equipped to explore and contribute to this rapidly growing area of machine learning.